INTERSECTION OBSERVER
BASICS:
What is Lazy Loading?
Lazy loading is a technique for waiting to load certain parts of a webpage like images until they are needed. Instead of loading everything all at once, known as “eager” loading, the browser does not request certain resources until the user interacts in such a way that the resources are needed. When implemented properly, lazy loading can speed up page load times.
Advantages of Lazy Loading:
1. Speeds up page load time: Since we are only requesting for content we require on a webpage on first load, we are reducing time taken to load the page. Which results in better performance like better SEO, higher conversion rate, improved user experience.
2. Saving in bandwidth: We are not loading “below the fold” images, so we are saving bandwidth required to deliver these images and time required to request and render these images.
What is Intersection Observer?
The Intersection Observer API lets code register a callback function that is executed whenever a particular element enters or exits an intersection with another element (or the viewport), or when the intersection between two elements changes by a specified amount.
Intersection Observer and usage:
The Intersection Observer API allows you to configure a callback that is called when either of these circumstances occur:
- A target element intersects either the device’s viewport or a specified element. That specified element is called the root element or root for the purposes of the Intersection Observer API.
- The first time the observer is initially asked to watch a target element.
let options = {
root: document.querySelector(“#scrollArea”),
rootMargin: “0px”,
threshold: 1.0,
};
let observer = new IntersectionObserver(callback, options);
Options parameter of intersection observer:
root: Element that is taken as reference for intersection with the target element. Defaults to browser viewport when not specified or provided as null.
rootMargin: Margin surrounding the root element. Can be given as CSS e.g. “10px 20px 30px 40px" (top, right, bottom, left).
threshold: Either a single number or an array of numbers which indicate at what percentage of the target’s visibility the observer’s callback should be executed
Callback function for intersection observer:
let callback = (entries, observer) => {
entries.forEach((entry) => {
// Each entry describes an intersection change for one observed
// target element:
// entry.boundingClientRect
// entry.intersectionRatio
// entry.intersectionRect
// entry.isIntersecting
// entry.rootBounds
// entry.target
// entry.time
});
};
USING INTERSECTION OBSERVER TO LAZY LOAD IMAGES:
Let’s take the observer we created above and apply it to images
const images = document.querySelectorAll(‘img’);
images.forEach(image => {
observer.observe(image);
});
//Intersection observer can’t observer more than
//- one element at a time so for a batch we need to iterate over it
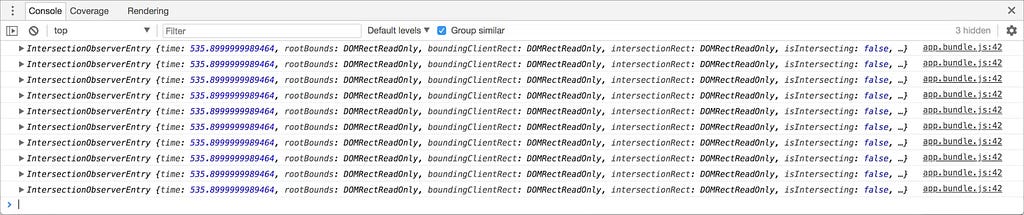
One of the most interesting property of Intersection observer is isIntersecting.
It can be used to find out whether the element being observed is entering the frame or leaving it. Based on this boolean value we can code our callback function to follow instructions.
isIntersecting : false — ->true Element is entering the frame.
isIntersecting : true — ->false Element is exiting the frame.
<img data-src=“https://phoo.com/foo.jpg”>
As you can see, the images should come without src tags: once a browser sees src attribute, it will start downloading that image right away that is opposite to our intentions. Hence we should not put that attribute on our images in HTML, and instead, we might rely on some data- attribute like data-src here.
Let’s write a code block to load the images as you scroll and when they intersect with the viewport. In this case our code will look something like:
const images = document.querySelectorAll(‘[data-src]’);
const options = { … };
let observer = new IntersectionObserver(function (entries, self) {
entries.forEach(entry => {
if (entry.isIntersecting)
{
preloadImage(entry.target)
self.unobserve(entry.target)//observer passed as self
}
});
}, options);
images.forEach(image => { observer.observe(image); });
Note. Instead of unobserve(event.target) we could also call disconnect(): it completely disconnects our IntersectionObserver and would not observe images anymore. This is useful if the only thing you care about is the first ever hit for your Observer.
IMPROVEMENT IN METRICS OBSERVED:
We were able to note significant gains on loading itemstack from search page:
1. Observed ~200KB reduction in initial HTML size.
2. Reduction of time taken to download initial content by ~250–300ms
3.Improvement in 75P LCP was also observed.
ADVANTAGES:
Lazy loading with Intersection Observer has several advantages in terms of metrics:
1. Improved page load performance: Lazy loading allows you to load only the necessary content or images when they become visible on the screen. This reduces the initial load time of the page, resulting in faster performance and improved user experience. It also helps reduce initial HTML size.
2. Reduced data usage: By loading content only when it is needed, lazy loading can significantly reduce data consumption. This is particularly beneficial for users with limited data plans or slower internet connections.
3. Increased conversion rates: Faster loading times and improved user experience can lead to higher conversion rates.
4. Lower bounce rates: Lazy loading can help reduce bounce rates, which is the percentage of users who leave a website after viewing only a single page. When users experience a faster loading time and can immediately see the content they are interested in, they are less likely to leave the site without exploring further.
5. Improved SEO performance: Lazy loading can also benefit SEO (Search Engine Optimization) efforts. Search engines prioritize websites that provide a good user experience, including fast loading times. By implementing lazy loading, you can improve your website’s loading speed, which can positively impact your search engine rankings and organic traffic.
DRAWBACKS:
While lazy loading with Intersection Observer provides many benefits, there are also a few drawbacks to consider:
1. Compatibility: One of the main drawbacks is that Intersection Observer is not supported in all browsers. It is a relatively new API and older browsers may not have built-in support for it.
2. Performance impact: While lazy loading can improve initial page load performance by deferring the loading of off-screen content, it can also have a negative impact on subsequent interactions. When the user scrolls or interacts with the page, there may be a delay as the unloaded content is fetched and rendered. This delay can negatively affect user experience, especially if there is a large amount of content to load.
3. Increased complexity: Implementing lazy loading with Intersection Observer requires additional code and logic compared to simply loading all content upfront. This can make the codebase more complex and harder to maintain. Additionally, if not implemented properly, it can lead to issues like content not loading when it should or unnecessary content loading when it shouldn’t.
4. SEO considerations: Lazy loading can have implications for search engine optimization (SEO). If search engine crawlers are unable to load and index lazy loaded content, it may impact the visibility and ranking of that content in search engine results. Considerations should be made to ensure that important content is accessible and crawlable by search engines.
5. Accessibility concerns: Lazy loading can also present accessibility challenges. Users with disabilities who rely on assistive technologies may have difficulty accessing and interacting with lazy loaded content if it is not properly implemented and signaled to assistive technologies.
Overall, while lazy loading with Intersection Observer can improve performance and user experience, it is important to carefully consider these drawbacks and address them appropriately in order to avoid any negative impact on your website or application.
References:
Intersection Observer API on MDN
Lazy Loading using Intersection Observer was originally published in Walmart Global Tech Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.
Article Link: Lazy Loading using Intersection Observer | by Sagar Bhatnagar | Walmart Global Tech Blog | Feb, 2024 | Medium