GraphQL Overview
Most people are familiar with REST API but probably not with GraphQL. GraphQL is an API Query language that supplies more flexibility and efficiency for fetching data, which can optimize RESTful API calls. It enables programmers to choose the types of requests they want to make and only fetch specific data as requested from server. Because of the benefits it brings, many of our applications within the Walmart Affiliate Team have adopted GraphQL instead of using REST.
GraphQL has three operation types: Query, Mutation and Subscription
Query and Mutation are mostly used. Query type is used to get specific data from server while mutation is used to change/update server-side data.
Example below:
GraphQL Request
query {
nodeDetail (input: { nodeId: “144”}) {
id
distance
name
address {
state
city
postalCode
}
}
}
GraphQL Response
{
“data”: {
“nodeDetail”: {
“id”: “144”,
“distance”: null,
“name”: “Walmart Supercenter”,
“address”: {
“state”: “AR”,
“city”: “FAYETTEVILLE”,
“postalCode”: “72704”
}
}
}
}
Arguments with values can be directly passed into the input field inside query, as shown above input: { nodeId: “144”}, and you can see that the query has the same shape as the result. The server will only fetch data that the client side is requesting for.
In the above example we write arguments inside the query string. But in most scenarios, arguments will be dynamic, so we use another field named variables to pass the dynamic argument. An example using variables is below:
query nodeDetail($input: NodeIdInput!){
nodeDetail(input: $input){
id
distance
name
address {
state
city
postalCode
}
}
}
Variables
{“nodeId”: “144”}
When making GraphQL API call from application, A typical payload of GraphQL is below
{
“query”: “SOME QUERY STRING”,
“variables”: {}
}
So, using the above example, it will be
"query": “query nodeDetail($input: NodeIdInput!){
nodeDetail(input: $input){
id
distance
name
address {
state
city
postalCode
}
}
}”,
“variables”: {“input”: {“nodeId”: “144”}}
Why Choose GraphQL
So now we have a basic understanding of GraphQL and let’s see what the major differences between REST and GraphQL.
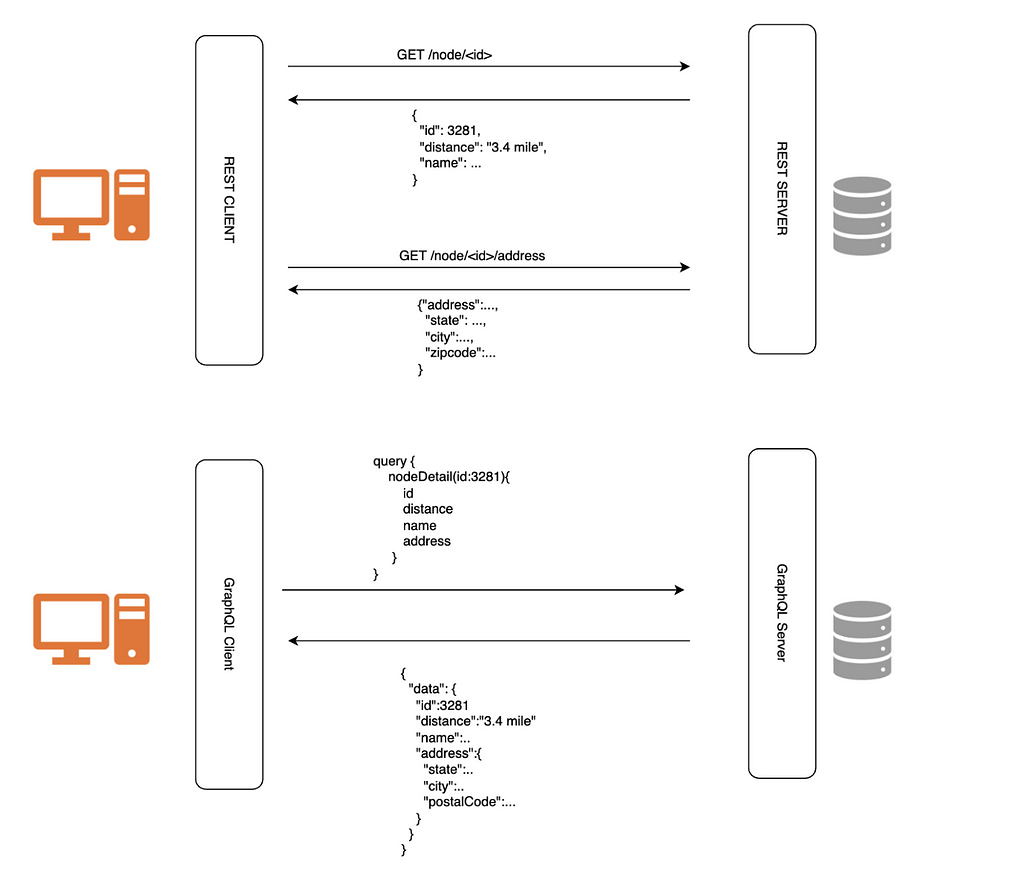
Multiple calls for REST, single call for GraphQL
From the graph below, you can see with a REST API, you would typically gather the data by querying multiple endpoints. For example, these could be first you need to query /node/<id> to get node information, then to get more address information, you would need to make call to the second endpoint /node/<id>/address. However, with GraphQL, with only a single query including concrete data requirements sent to the server, the GraphQL server will then respond with a JSON object where these requirements are fulfilled.
One example of our Affiliate Team applications is store service locator API which is used by 40+ partners to get Walmart store related information. The service had to call multiple internal services to get store information i.e., names, distances, etc., which could reach around 1.5 seconds 95th percentile latency, plus there were multiple dependencies and unnecessary data we don’t need from response. After switching to single internal GraphQL API, we can specify only the data we need, the latency reduced 10% and there’s less dependency as well.
Over and under fetching for REST API, specific data from GraphQL
Because for REST, the only way for clients to retrieve data from server is by hitting endpoints that return fixed structured data. So, it’s hard to design the API to provide clients with their specific data requirements. The result could be it over fetches data from server that clients need, e.g., client only wants to get user’s name and email address information but may end up with getting more such as shipping address or billing info which are unnecessary. Or it could end up with under-fetching such as user needs both address of the store and basic store information like name and distance but have to make /node/<id> to get basic store info first then an added API to get store address by making /node/<id>/address.
Flexibility and Efficiency
As for performance, GraphQL is better than REST in two aspects. REST API had rigid data structure and designed only to get back fixed data whenever they get hit, GraphQL is more flexible given the fact that you would only request desired data format. GraphQL is friendly to low-resourced devices such as mobile apps and IoT devices that can be added to network without worrying about new endpoints and enhancements to data retrieval and processing. Also, with GraphQL, you could get data with one single call while REST API will over or under fetch data. Given these facts, GraphQL could be more flexible and more efficient.
Comparison between GraphQL vs REST
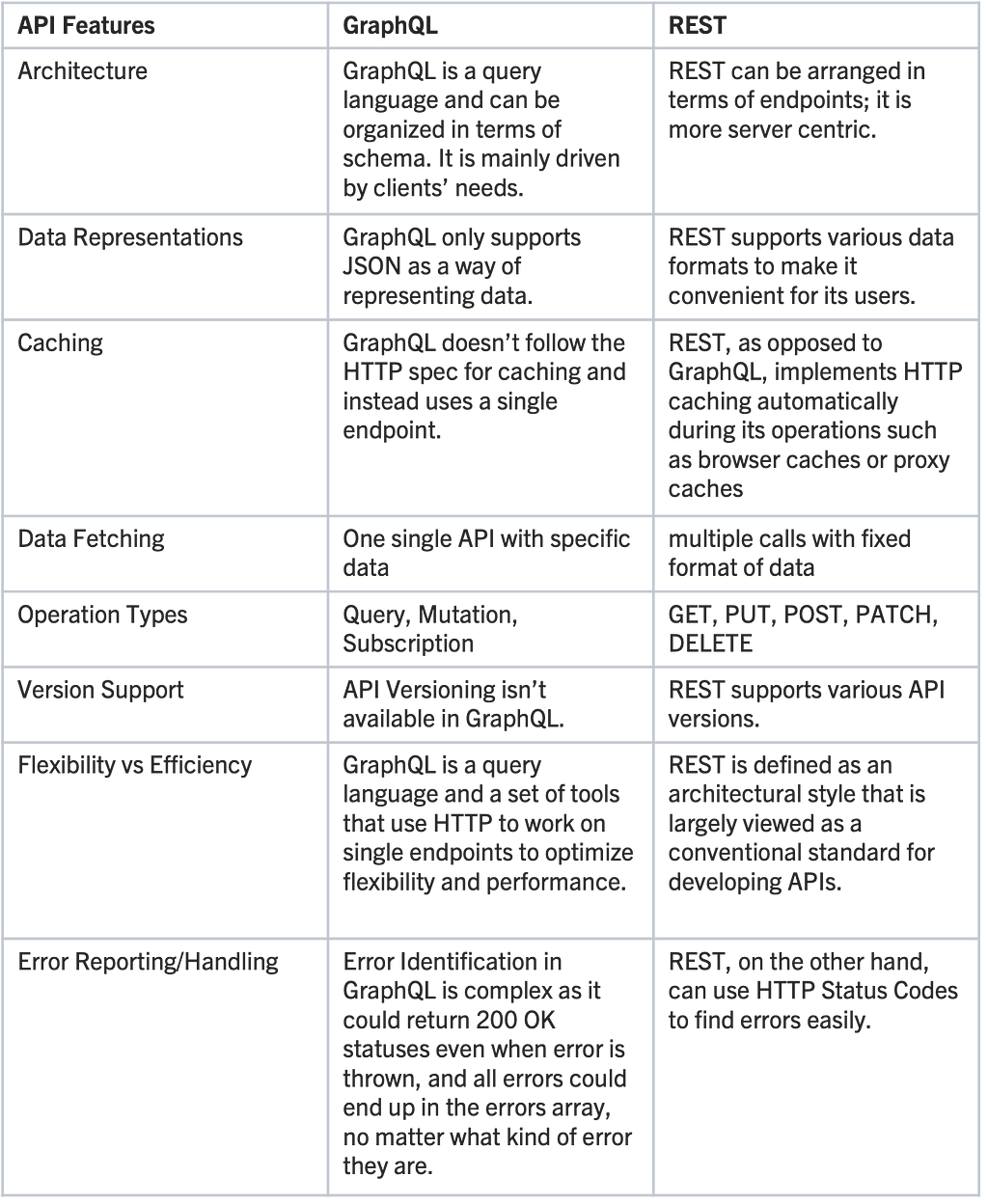
When to use REST
Even though GraphQL is awesome, but REST will be a better choice in some of the use cases
Caching Mechanism
Nowadays, every browser supplies an implementation of an HTTP cache to easily find if two resources are the same to avoid re-fetching. When using GraphQL there is no way to get a globally unique identifier for a given object because we use the same URL for all the requests. To have cache on GraphQL you have to setup your own. But REST can achieve caching.
Service to Service Communications
When it comes to service-to-service communication, REST is more popular due to its simplicity; services communicate directly and synchronously with each other over HTTP, without the need for any added infrastructure, which gives developers the flexibility to custom-build APIs for specific business requirements.
Monitoring and Error Reporting
You can build a monitoring and alerting system based on response status of REST API response. But for GraphQL, it could return 200 OK statuses even when error is thrown, and all errors could end up in the errors array, no matter what kind of error they are, so it’s not straightforward to find an error. E.g. An error response from GraphQL could be below:
HTTP 200 OK
{errors: [ {message: ‘Internal Server Error’}]}
Conclusion
This article mainly gives a brief introduction of GraphQL and talks about the major factors for the GraphQL vs REST to help you understand them better. In general, both are good in their way so selecting GraphQL or REST depends on the use cases. GraphQL offers many improvements over REST with its declarative and flexible structure, however, it’s not suitable in all cases. For instance, if you are planning to develop mobile apps GraphQL is preferred considering bandwidth usage, while REST should be chosen if your system requires a more robust and mature API.
GraphQL vs REST was originally published in Walmart Global Tech Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.
Article Link: GraphQL vs REST. GraphQL Overview | by supergirl | Walmart Global Tech Blog | Feb, 2022 | Medium