Binary Ninja’s extensibility allows for powerful customizations, one of which is the ability to tailor how data is presented in Linear View. This capability is primarily provided by the DataRenderer
class (C++, Python). In this post, we’ll delve into how to leverage a DataRenderer
to create custom representations for specific types of data, enhancing the clarity and utility of Binary Ninja’s interface for reverse engineering tasks.
Understanding DataRenderers
The DataRenderer
class in Binary Ninja enables developers to define custom visual representations for data types in Linear View. This mechanism is particularly useful when the default rendering does not meet specific needs or when a more domain-specific visualization can provide clearer insights into the data’s structure and meaning.
Key Components of a DataRenderer
-
is_valid_for_data
Method: This method determines if the custom renderer is applicable for a given type of data, based on the address (addr
) and context (context
). The context is a sequence of Type objects representing the chain of nested objects being displayed. -
get_lines_for_data
Method: This method generates the visual representation for the data, returning a list ofDisassemblyTextLine
objects. Each object represents a single line of output in the Linear View. The method allows for the integration of custom text or graphical elements into the view.
Registering DataRenderers
To make a DataRenderer
active, it must be registered with Binary Ninja’s core. This can be done using either register_type_specific
or register_generic
. Type-specific renderers have precedence over generic ones, allowing for fine-grained control over the rendering of certain data types.
Example: Customizing Display for COM GUIDs
Consider a scenario like reverse engineering a COM library: A whole series of GUIDs will be present and we’ll want to display them in a more human-readable format. A DataRenderer
is the perfect solution for this.
Defining a Custom Renderer
Here’s a custom DataRenderer in python – it mirrors one implemented in our core when we released our COMpanion plugin. There’s no need to use this exact plugin in recent versions, but it’s provided as a useful example.
class GuidDataRenderer(DataRenderer): def __init__(self): super(GuidDataRenderer, self).__init__()
def is_valid_for_data(self, view, addr, type, context): # Equivalent of checking the platform if not view.platform: return False # Check if the type is a structure and named "_GUID" if type.type_class == TypeClass.StructureTypeClass: ntr = type.registered_name if ntr and ntr.name == "_GUID": # Ensure the address range for a GUID is valid if view.is_valid_offset(addr) and view.is_valid_offset(addr + 16): return True return False def get_lines_for_data(self, view, addr, type, prefix, width, context): result = [] line = DisassemblyTextLine(addr, prefix) result.append(line) line.tokens = [] reader = BinaryReader(view) reader.seek(addr) data1 = reader.read32() data2 = reader.read16() data3 = reader.read16() dataEnd = reader.read64be() data4 = (dataEnd >> 48) & 0xffff data5 = dataEnd & 0x0000FFFFFFFFFFFF guid_str = f"{data1:08x}-{data2:04x}-{data3:04x}-{data4:04x}-{data5:012x}" line.tokens.append(InstructionTextToken(InstructionTextTokenType.TextToken, " [Guid(\"")) line.tokens.append(InstructionTextToken(InstructionTextTokenType.StringToken, guid_str)) line.tokens.append(InstructionTextToken(InstructionTextTokenType.TextToken, "\")]")) result.append(DisassemblyTextLine(addr, line.tokens)) # Check for type name by GUID and add it to the display if type_name := view.get_type_name_by_guid(guid_str): line = DisassemblyTextLine(addr) line.tokens.append(InstructionTextToken(InstructionTextTokenType.TextToken, " interface ")) line.tokens.append(InstructionTextToken(InstructionTextTokenType.TypeNameToken, type_name)) result.append(line) return result
GuidDataRenderer().register_type_specific()
Activating the Renderer
GuidDataRenderer().register_type_specific()
By registering the renderer as type-specific, we ensure that it will be used for _GUID
structures, overriding the generic structure renderer.
Results
With the custom GuidDataRenderer
in place, the GUIDs in Linear View will now be displayed in a more human-readable format, including the GUID string and the corresponding interface name. This enhanced visualization can significantly improve the reverse engineering process by providing more context and clarity around the data being analyzed.
Here’s a before and after image of the renderer being enabled:
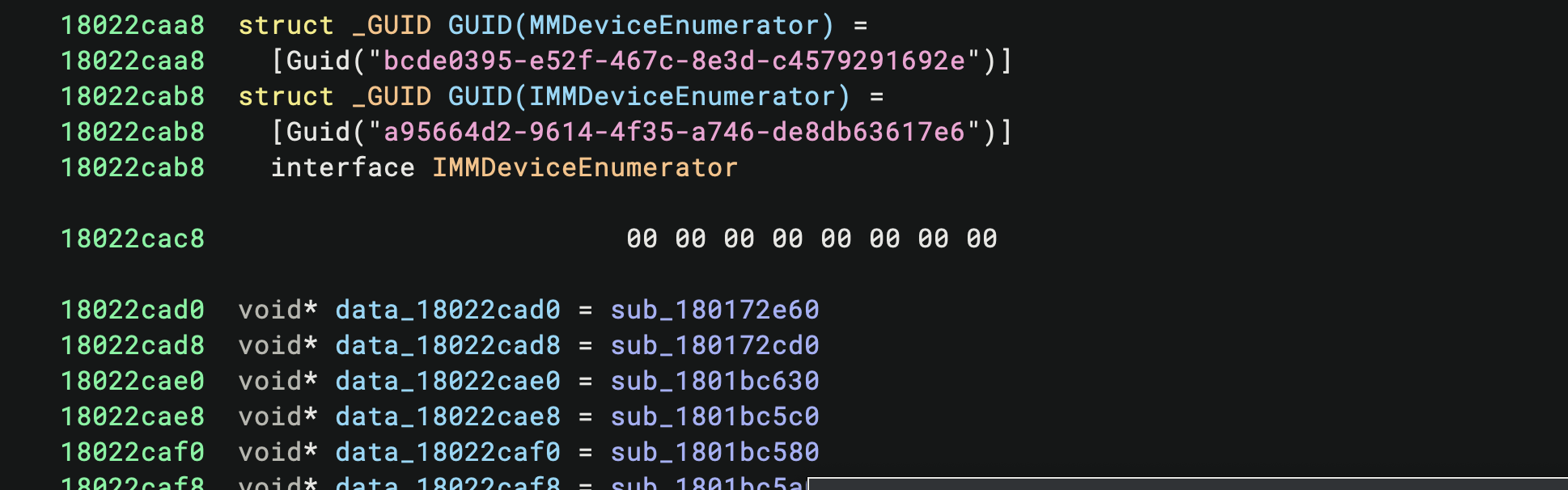
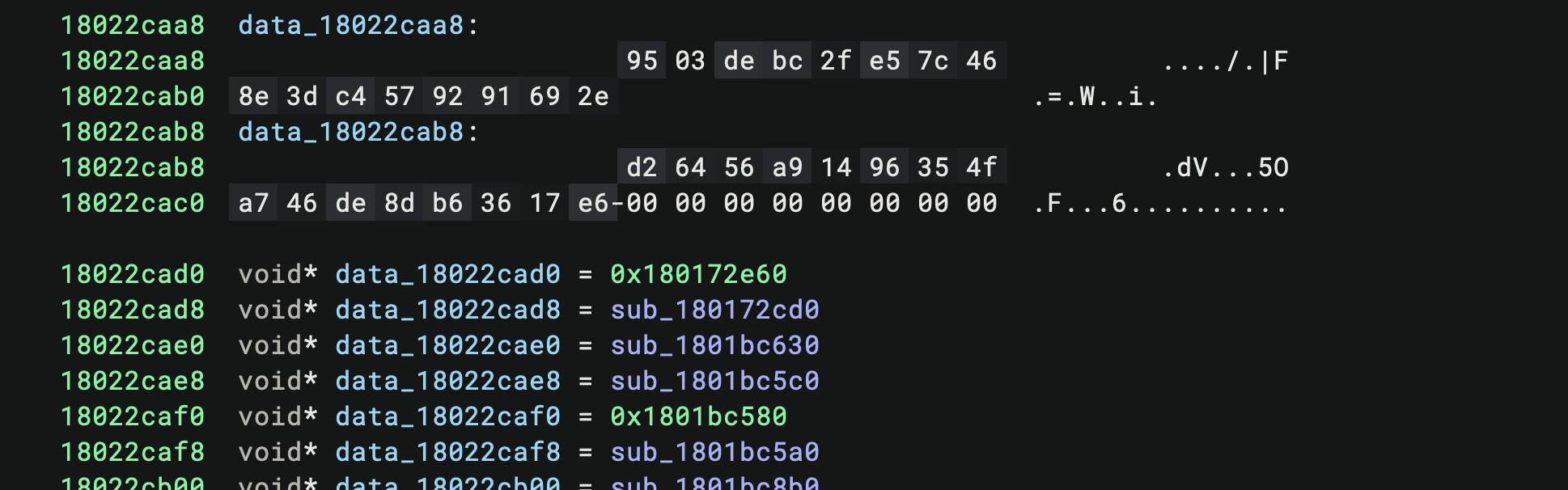
Article Link: Binary Ninja - Customizing Data Display in Binary Ninja with a DataRenderer